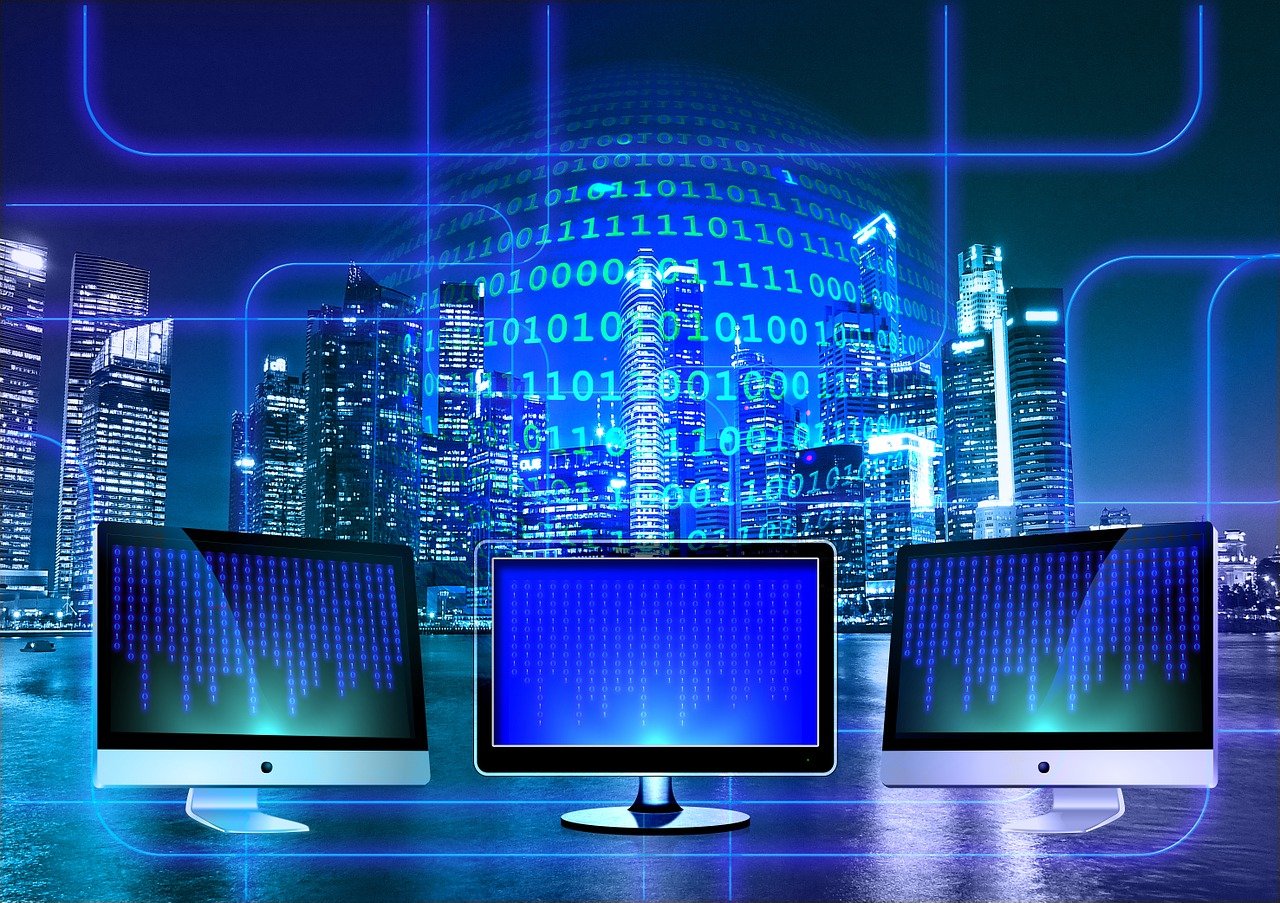
What is the Fetch API in JavaScript?
The Fetch API in JavaScript is a modern interface that enables developers to make HTTP requests to servers from web browsers. It provides a more efficient and powerful way of making HTTP requests than traditional methods like XMLHttpRequest. The Fetch API is primarily designed to replace the older XMLHttpRequest, offering a more straightforward and flexible way to handle HTTP requests, making it easier for developers to work with APIs and fetch data from servers.
Understanding Fetch API Basics
What Does Fetch API Do?
The Fetch API allows developers to make network requests using a simple and intuitive syntax. It provides a cleaner and more modern approach compared to traditional methods like XMLHttpRequest, making it easier for developers to consume resources asynchronously without the need for additional libraries.
Why Use Fetch Over Other Methods?
Compared to XMLHttpRequest, the Fetch API offers a more powerful and flexible feature set, cleaner and simpler syntax, and uses Promises for easier chaining and async/await without callbacks. This makes it an attractive choice for developers looking for a modern alternative to XMLHttpRequest.
Key Features of the Fetch API
Simple to Use
The Fetch API provides a straightforward and easy-to-use interface for making network requests. Its simplicity makes it accessible even for beginners who are just starting with web development.
Works With Promises
One of the key features of the Fetch API is its seamless integration with Promises. This allows developers to write asynchronous code in a more readable and maintainable way, improving the overall efficiency of handling network requests.
By leveraging the power of the Fetch API, developers can streamline their code, improve performance, and create more responsive web applications.
How to Make Your First Fetch Request
Now that you have a basic understanding of the Fetch API and its key features, it’s time to dive into making your first fetch request. This section will guide you through setting up your JavaScript project, writing your first fetch request, and providing examples of different types of Fetch API requests.
Setting Up Your JavaScript Project
Tools You Need
Before you start working with the Fetch API, ensure that you have a text editor or an integrated development environment (IDE) installed on your computer. Popular choices include Visual Studio Code, Sublime Text, or Atom. These tools provide a user-friendly interface for writing and organizing your code effectively.
Creating a Simple HTML Page
To practice making fetch requests, create a simple HTML page where you can write and test your JavaScript code. Start by creating an HTML file with the necessary structure, including the <html>
, <head>
, and <body>
tags. Within the <body>
tag, you can include a button or any other interactive element that triggers the fetch request when clicked.
Writing Your First Fetch Request
The Basic Structure of a Fetch Request
To make a fetch request in JavaScript, use the fetch()
function followed by the URL of the resource you want to retrieve. This function returns a Promise that resolves to the Response to that request. Here’s an example of how to make a simple GET request using the Fetch API:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Handling the Response
Once you’ve made a fetch request, it’s essential to handle the response appropriately. The then()
method is used to process the resolved value (the response) once it’s available. In this example, we’re converting the response to JSON format using response.json()
. Additionally, we’re using catch()
to handle any errors that may occur during the request.
Examples of Fetch API Requests
Getting Data From a Website
You can use the Fetch API to retrieve data from external websites or APIs. For instance, if you want to display weather information on your website, you can make a fetch request to a weather API and then use the received data to update your web page dynamically.
Sending Data to a Server
In addition to fetching data from servers, the Fetch API also allows you to send data via HTTP methods like POST or PUT. This enables you to interact with server-side resources by sending user input or other relevant data for processing.
By practicing these examples and experimenting with different types of requests, you’ll gain valuable experience in utilizing the Fetch API effectively within your JavaScript projects.
Tips for Using the Fetch API Like a Pro
When working with the Fetch API in JavaScript, it’s essential to employ best practices and advanced techniques to ensure efficient and reliable network requests. This section will delve into handling errors gracefully and making requests faster and more efficient.
Handling Errors Gracefully
Proper error handling ensures that your application remains stable and user-friendly. When utilizing the Fetch API, it’s crucial to check for response success and handle potential issues such as network errors or unexpected response data. Ensuring that the application can gracefully handle these issues is crucial for providing a smooth user experience.
One of the key aspects of error handling with the Fetch API is checking for response success. By consistently verifying the success of responses, you can ensure that your application remains reliable even when things don’t go as planned. This approach aligns with best practices for making robust applications, where recognizing and handling errors efficiently is paramount.
Handling errors is consistent across all four fetch methods, ensuring that any fetch-related errors are captured and reported. Although the JavaScript Fetch API provides an ideal way to make network requests, it is very necessary to handle potential errors that occur during the process.
What to Do When Things Go Wrong
While the Fetch API makes it easy to catch network errors, handling errors in the response (like a 404 status) requires additional logic. The project could benefit from more robust error handling in such scenarios, ensuring a seamless experience for users even when unexpected issues arise.
Making Your Requests Faster and More Efficient
In addition to error handling, optimizing your fetch requests for speed and efficiency is crucial for delivering responsive web applications. Utilizing advanced features like Async/Await and caching responses can significantly enhance the performance of your fetch requests.
Using Async/Await allows you to write asynchronous code in a more readable and maintainable way. By leveraging this feature, you can streamline your codebase, making it easier to manage complex asynchronous operations while maintaining clarity in your code structure.
Caching responses is another powerful technique for improving request efficiency. By storing previously fetched data locally, you can reduce redundant network requests, leading to faster load times and improved overall performance.
By incorporating these advanced techniques into your development workflow, you can elevate your proficiency in using the Fetch API effectively within your JavaScript projects while optimizing speed and reliability.
Wrapping Up
As you conclude your journey into mastering the Fetch API in JavaScript, it’s essential to remember that practice makes perfect. By experimenting with different APIs and building small projects, you can solidify your understanding and application of the Fetch API’s capabilities.
Practice Makes Perfect
Experiment With Different APIs
Embracing various APIs allows you to explore diverse data sources and functionalities, broadening your exposure to different web services. Consider integrating the Fetch API with weather, news, or social media APIs to gain hands-on experience in working with a range of data formats and endpoints.
Build Small Projects
Constructing small-scale applications that utilize the Fetch API enables you to apply your knowledge in real-world scenarios. Whether it’s creating a weather app, a news aggregator, or a user authentication system, these projects provide valuable opportunities to refine your skills and showcase the versatility of the Fetch API.
Keep Learning and Exploring
Continuing your learning journey is crucial for staying updated with the latest advancements in web development. Here are some resources for further learning and connecting with like-minded individuals:
Resources for Further Learning
- Online tutorials and courses on JavaScript and web APIs can deepen your understanding of the Fetch API’s intricacies.
- Books such as “JavaScript & jQuery: The Missing Manual” by David Sawyer McFarland offer comprehensive insights into modern web development practices.
- Platforms like MDN Web Docs provide detailed documentation on the Fetch API, serving as a valuable reference during your coding endeavors.
Joining Communities
Engaging with developer communities through forums, meetups, or online groups fosters collaboration and knowledge sharing. Platforms like Stack Overflow and GitHub Discussions offer spaces where you can seek advice, share experiences, and contribute to discussions related to JavaScript and web development.
By immersing yourself in these resources and communities, you can stay motivated, informed, and inspired as you continue honing your skills in utilizing the Fetch API effectively within your JavaScript projects.