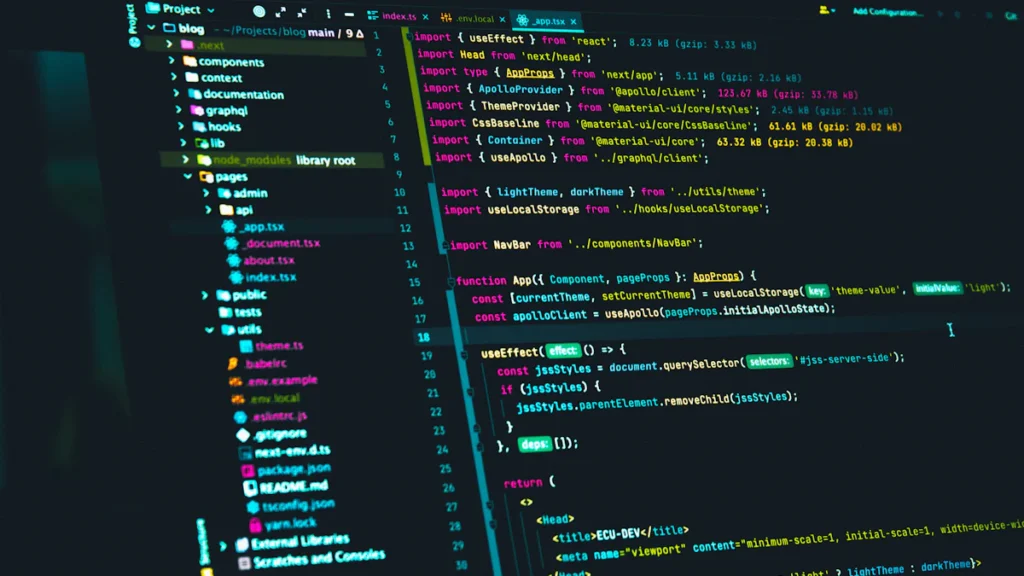
What Are JavaScript Objects?
The Basics of JavaScript
JavaScript is a widely used programming language that plays a crucial role in web development. JavaScript is known for its versatility and ability to create interactive and dynamic elements on websites. It is a client-side scripting language, meaning it runs on the user’s web browser rather than the server, allowing for real-time interactions without the need to reload the entire page.
One of the key reasons why JavaScript is so popular is its compatibility with various platforms and its extensive library of frameworks and libraries. According to recent statistics, approximately 98.6% of all websites utilize JavaScript, showcasing its dominance in the web development landscape. Furthermore, over 78% of professional developers and web development professionals use JavaScript in their projects, highlighting its widespread adoption within the industry.
Exploring Objects in JavaScript
In JavaScript, an object is a standalone entity, with properties and type. These objects are used to store collections of data and more complex entities. But what exactly makes an object? An object in JavaScript consists of key-value pairs that represent attributes or characteristics of the object. This allows for a structured organization of data, making it easier to manage and manipulate information within a program.
To better understand objects in JavaScript, let’s consider some examples of simple objects. For instance, an object representing a car could have properties such as “make,” “model,” “year,” and “color.” Each property would have a corresponding value, providing a comprehensive representation of the car within the program.
By exploring these fundamental concepts, we can gain a deeper understanding of how objects function within the context of JavaScript programming.
Understanding Object Constructors in JavaScript
Now that we have a foundational understanding of JavaScript objects, let’s delve into the concept of object constructors.
What Are Object Constructors?
In JavaScript, an object constructor is a blueprint for creating objects with similar properties and methods. It serves as a template that defines how each instance of an object should be structured. The role of constructors in making objects is akin to a master plan for constructing buildings; they provide the specifications and guidelines for creating new instances of a particular type of object.
Object constructors work by defining the structure and behavior of an object through a function. When this function is invoked with the new
keyword, it creates a new instance of the object based on the defined blueprint. This process allows for the efficient creation of multiple objects with consistent attributes and methods.
Types of Constructors in JavaScript
Built-in Constructors
JavaScript provides several built-in constructors that are readily available for creating common types of objects, such as strings, arrays, dates, and more. These built-in constructors offer predefined templates for creating instances of these fundamental data types, simplifying the process of working with these objects within a program.
Custom Constructors
In addition to built-in constructors, developers can create custom constructors to define their own object types with specific properties and methods tailored to their unique requirements. Custom constructors empower developers to encapsulate related functionality within distinct object types, promoting modularity and reusability within their codebase.
By understanding these different types of constructors in JavaScript, developers can leverage both built-in and custom solutions to efficiently create and manage diverse sets of objects within their applications.
Step-by-Step Guide to Creating Objects with Constructors
Now that we have a solid understanding of JavaScript objects and object constructors, let’s explore the step-by-step process of creating objects using constructors.
Preparing to Use Object Constructors
Before we dive into creating objects with constructors, it’s essential to ensure that our coding environment is set up correctly. This involves having a text editor or an integrated development environment (IDE) where we can write and execute our JavaScript code. Additionally, having a web browser to run and test our code is crucial for visualizing the output of our programs.
Once our coding environment is ready, we can begin by familiarizing ourselves with the basic syntax for defining an object constructor in JavaScript.
Setting Up Your Coding Environment
To set up your coding environment, you can choose from popular text editors such as Visual Studio Code, Sublime Text, or Atom. These editors provide features like syntax highlighting and auto-completion, making it easier to write and debug JavaScript code. Alternatively, you may opt for an IDE like WebStorm or Brackets, which offer more comprehensive tools specifically designed for web development.
After selecting your preferred text editor or IDE, ensure that you have a modern web browser installed on your system. Browsers like Google Chrome, Mozilla Firefox, or Microsoft Edge are commonly used for testing and running JavaScript applications.
Basic Syntax for a Constructor
In JavaScript, the basic syntax for defining an object constructor involves creating a function that will serve as the blueprint for generating new instances of objects. The function typically uses PascalCase naming convention to distinguish it as a constructor function.
Here’s an example of the basic syntax for a constructor:
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
In this example, we’ve defined a constructor function called Car
that takes parameters for “make,” “model,” and “year.” Inside the function body, we use the this
keyword to assign values to the properties of the newly created object based on the provided arguments.
Creating Your First Object with a Constructor
With our coding environment set up and the basic syntax understood, let’s proceed with creating our first object using a constructor in JavaScript.
Writing the Constructor Function
To create an instance of an object using a constructor function, we simply invoke the function using the new
keyword followed by its name. This action instantiates a new object based on the blueprint defined within the constructor function.
Let’s consider how we can use our Car
constructor to create an instance representing a specific car:
let myCar = new Car('Toyota', 'Camry', 2022);
In this example, we’ve used the Car
constructor to create an object named myCar
, which represents a Toyota Camry manufactured in 2022.
Using the Constructor to Make an Object
By invoking the Car
constructor with different arguments, we can create multiple instances representing various cars with distinct attributes. This demonstrates how constructors enable us to efficiently produce numerous objects following a consistent structure defined by their respective blueprints.
Adding Properties and Methods
As we become more proficient in utilizing object constructors in JavaScript, we can enhance our objects by adding additional properties and methods tailored to specific requirements.
Enhancing Objects with More Features
We can expand upon our initial Car
example by incorporating additional properties such as “color,” “mileage,” or “fuelType.” These enhancements allow us to capture more detailed information about each car within our program.
Examples of Objects with Properties and Methods
Furthermore, beyond simple data properties like those mentioned above, objects in JavaScript can also contain methods—functions associated with them that enable them to perform actions or computations. For instance…
Wrapping Up
As we conclude our exploration of JavaScript object constructors, it’s essential to review the key takeaways from our discussion and consider the practical significance of utilizing object constructors in programming.
Reviewing What We’ve Learned
Key Takeaways
Throughout this guide, we’ve learned that Object Constructors serve as blueprints for creating objects with consistent properties and methods. They enable developers to define custom object types tailored to specific requirements, promoting modularity and reusability within their codebase. By leveraging both built-in and custom constructors, programmers can efficiently manage diverse sets of objects within their applications.
Furthermore, we’ve gained a deeper understanding of how JavaScript objects are structured using key-value pairs to represent attributes or characteristics. This structured organization facilitates the management and manipulation of data within a program, enhancing the overall efficiency of JavaScript applications.
Why Object Constructors Are Useful
Object constructors are invaluable tools in JavaScript development due to their ability to streamline the creation of multiple instances of objects with consistent structures. They promote code reusability by encapsulating related functionality within distinct object types, leading to more maintainable and scalable applications. Additionally, object constructors contribute to the modular design of programs, allowing developers to manage complex data structures effectively.
Practice Makes Perfect
Ideas for Further Practice
To reinforce your understanding of Object Constructors in JavaScript, consider engaging in the following practice exercises:
- Create a custom constructor for a “Book” object that includes properties such as “title,” “author,” and “genre.”
- Implement methods within an existing constructor to perform specific actions based on the object’s properties.
- Explore advanced use cases for object constructors by incorporating inheritance and prototype-based programming concepts.
Encouragement to Keep Learning
As you continue your journey in mastering JavaScript development, remember that practice is key to solidifying your skills. Embrace challenges as opportunities for growth, and stay curious about new techniques and best practices in object-oriented programming. By consistently honing your abilities with object constructors, you’ll enhance your proficiency in building robust and scalable applications.