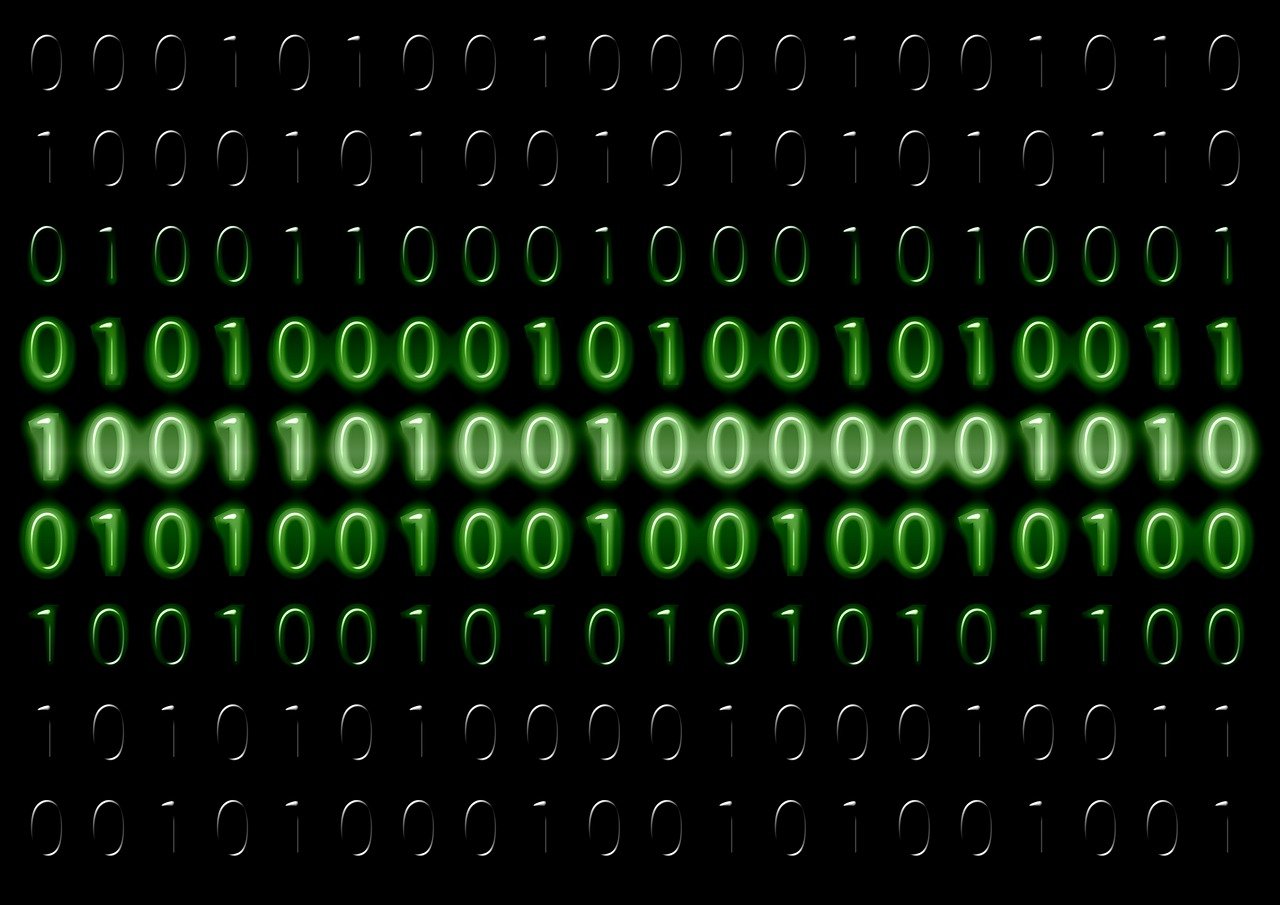
Understanding Prime Numbers and Their Importance
Prime numbers play a crucial role in various fields, including mathematics, cryptography, and computer science. But what exactly is a prime number and why do they matter? Let’s delve into the significance of prime numbers and their impact on programming.
What is a Prime Number?
Definition and Examples
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. For example, 2, 3, 5, 7, and 11 are all prime numbers because they are only divisible by 1 and themselves. This unique characteristic makes prime numbers fundamental building blocks of all positive integers.
Why Prime Numbers Matter
Prime numbers are not just abstract mathematical concepts; they have practical implications in real-world applications. They are extensively used in modern cryptography, including the RSA algorithm and public/private key encryption. The use of prime factors of large numbers for encryption ensures secure communication by making it computationally infeasible to decrypt messages without the correct keys.
The Role of Prime Numbers in Programming
Cryptography and Security
In programming, prime numbers are integral to ensuring data security. They form the basis for cryptographic algorithms that protect sensitive information during transmission and storage. By leveraging the unique properties of prime numbers, programmers can develop robust encryption methods to safeguard digital communications.
Algorithms and Efficiency
Furthermore, prime numbers contribute to the efficiency of various algorithms used in programming. Their distinct properties enable the development of optimized algorithms for tasks such as factorization, which is essential for solving problems in number theory and cryptography.
By understanding the significance of prime numbers in both theoretical mathematics and practical applications like programming, we gain valuable insights into their pervasive influence across diverse domains.
Diving Into Python: Basics You Need to Know
Python has emerged as a popular choice for developing a prime number checker due to its simplicity, versatility, and educational value. Let’s explore why Python is the ideal programming language for implementing a prime number checker and delve into key concepts that beginners should be familiar with.
Why Python for Your Prime Number Checker?
Python‘s simplicity and versatility make it an excellent choice for creating a prime number checker. Unlike other programming languages, Python emphasizes readability and straightforward syntax, allowing developers to focus on problem-solving rather than intricate code structures. This standout feature makes Python an attractive option for full-stack web development, where efficiency and clarity are paramount.
Moreover, the educational value of Python cannot be overstated. Its intuitive nature and extensive documentation make it accessible to beginners, enabling them to grasp fundamental programming concepts while working on practical projects like a prime number checker.
Key Python Concepts for Beginners
Variables and Data Types
In Python, variables serve as containers for storing data values. They play a crucial role in programming by enabling developers to manipulate and process information efficiently. Understanding different data types, such as integers, floats, strings, and booleans, is essential when building a prime number checker. For instance, variables can store the input number to be checked and the result of the primality test.
Loops and Conditions
Loops are fundamental in programming as they allow repetitive execution of code based on certain conditions. In the context of a prime number checker, loops can be utilized to iterate through a range of numbers to determine their primality. Additionally, conditions, represented by keywords like if
, else
, and elif
, enable developers to implement logic for evaluating whether a number meets the criteria of being prime.
By familiarizing themselves with these key concepts in Python, aspiring programmers can lay a solid foundation for creating efficient and functional programs like a prime number checker.
Crafting Your Prime Number Checker in Python
Now that we understand the significance of prime numbers and have explored the basics of Python, it’s time to craft our very own prime number checker program. This section will guide you through setting up your Python environment and writing the code for the prime number checker.
Setting Up Your Python Environment
Installing Python
Before diving into coding, ensure that Python is installed on your system. You can download the latest version of Python from the official website and follow the installation instructions provided. Once installed, you’ll have access to a powerful and versatile programming language for developing various applications, including our prime number checker.
Using a Text Editor or IDE
Next, choose a suitable text editor or integrated development environment (IDE) for writing your Python code. Popular options include Visual Studio Code, PyCharm, Sublime Text, and Atom. These tools offer features such as syntax highlighting, auto-indentation, and integrated terminal support, enhancing your coding experience.
Writing the Prime Number Checker Code
Step-by-Step Code Walkthrough
Let’s begin by defining the logic for our prime number checker. We’ll create a function that takes an input number and determines whether it is a prime number or not. Here’s a step-by-step breakdown of the code:
- Input: Receive an integer input from the user.
- Validation: Check if the input is a positive integer greater than 1.
- Primality Test: Implement a loop to check divisibility of the input number by all integers up to its square root.
- Result: Display whether the input number is prime or not based on the divisibility test.
Below is an example of how this logic can be implemented in Python:
def prime_number_checker(num):
if num <= 1:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
# Input from user
number = int(input("Enter a positive integer: "))
# Perform primality test
if prime_number_checker(number):
print(f"{number} is a prime number.")
else:
print(f"{number} is not a prime number.")
Testing and Debugging Your Program
After writing the code, it’s essential to thoroughly test it with different inputs to ensure its accuracy and reliability. Consider scenarios with both prime and non-prime numbers to validate the correctness of your program. Additionally, if any errors or unexpected behavior occur during testing, utilize debugging techniques such as printing intermediate values and using breakpoints to identify and resolve issues effectively.
By following these steps and leveraging Python’s simplicity and flexibility, you can create a robust prime number checker program that demonstrates both mathematical concepts and programming skills.
Wrapping Up
As we conclude our exploration of prime numbers and the creation of a prime number checker in Python, it’s essential to reflect on the key takeaways from this journey and consider the potential challenges that may arise when working on similar programming projects.
What You’ve Learned Today
Key Takeaways
- Prime numbers are fundamental building blocks of all positive integers, playing a crucial role in various fields such as mathematics, cryptography, and computer science.
- Understanding prime numbers is essential for modern security and cryptography, as they are used extensively in encryption algorithms like RSA to protect sensitive information.
- Python’s simplicity and versatility make it an excellent choice for developing a prime number checker, offering accessibility to beginners and efficiency to experienced programmers.
Potential Challenges and How to Overcome Them
When delving into Python programming and exploring mathematical concepts like prime numbers, you may encounter challenges such as:
- Algorithm Optimization: Developing efficient algorithms for primality testing can be complex. To overcome this challenge, leverage Python’s libraries like SymPy or NumPy that offer optimized functions for handling prime numbers.
- Error Handling: Dealing with user input errors or unexpected behavior in the program requires robust error-handling techniques. Utilize Python’s exception handling mechanisms to gracefully manage errors and maintain program stability.
- Understanding Cryptographic Concepts: Implementing cryptographic principles using prime numbers demands a solid understanding of encryption techniques. Engage with online resources and tutorials focused on cryptography to enhance your knowledge in this area.
By acknowledging these potential challenges and proactively seeking solutions, you can navigate through the intricacies of Python programming while gaining a deeper comprehension of prime numbers’ significance in computational security.
Next Steps in Your Python Journey
As you continue your Python journey beyond creating a prime number checker, consider exploring further learning resources and engaging in exciting project ideas that align with your interests and aspirations.
Further Learning Resources
- Online courses on advanced Python programming techniques
- Books covering cryptography fundamentals and applications
- Community forums for discussing prime number algorithms and encryption practices
Project Ideas to Explore
- Developing a secure messaging application using public/private key encryption
- Creating a web-based tool for generating large prime numbers
- Contributing to open-source projects related to mathematical computations in Python
Embarking on these next steps will not only enhance your proficiency in Python but also deepen your understanding of how prime numbers intersect with real-world applications in cybersecurity and beyond.