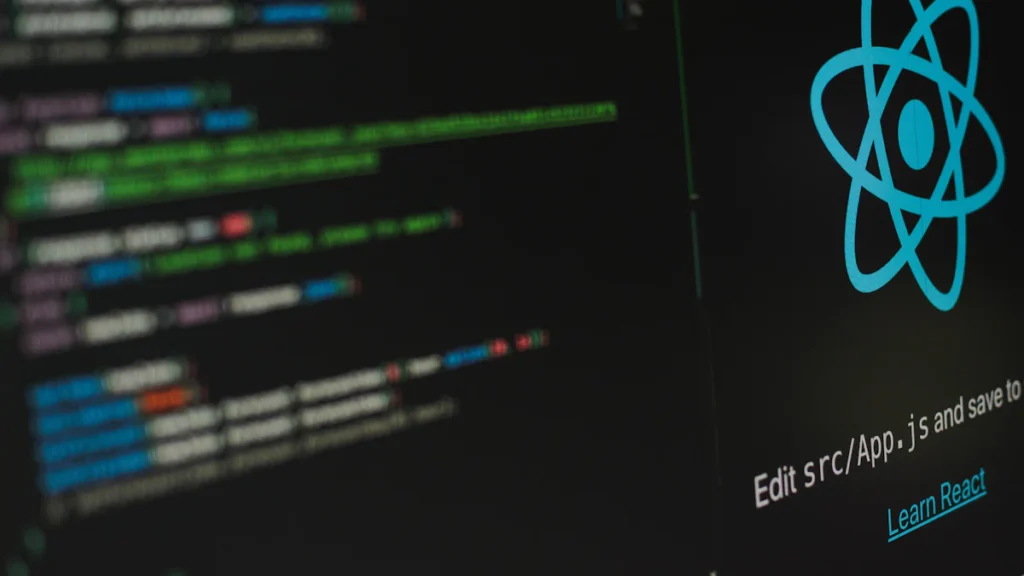
Getting Started with Node.js
If you’re new to programming, Node.js is an excellent platform to begin your journey. It’s widely favored by developers and business owners, with 42.65% of the votes and 36.42% of professional developers using it for various projects. Additionally, it powers over 6.3 million websites, making it a significant player in the web development space.
Why Node.js is Awesome for Beginners
Node.js is known for its ease of learning and versatile use cases, making it an ideal choice for beginners. With its simple and straightforward syntax, aspiring developers can quickly grasp the fundamentals of JavaScript and server-side scripting.
- Easy to Learn: Learning Node.js becomes significantly easier if already familiar with JavaScript. It utilizes JavaScript for both server-side and client-side scripting, allowing a seamless transition between frontend and backend development.
- Versatile Use Cases: 85% of developers use Node.js primarily for developing web applications, while 43% use it to build enterprise applications. Its scalability, simplicity, and unified language for backend and frontend development make it a versatile tool for various projects.
Setting Up Your Node.js Environment
To get started with Node.js, you’ll need to set up your development environment:
Installing Node.js
Begin by installing Node.js on your machine. You can download the installer from the official website or use a package manager like npm to install it seamlessly.
Writing Your First Node.js Script
Once installed, you can start writing your first Node.js script. The platform provides an array of resources and tutorials to guide you through this process.
How To Check if a String Contains a Specific Word in Node.js
Understanding Strings in JavaScript
Strings are a fundamental data type in JavaScript, representing a sequence of characters. In Node.js, strings are commonly used for storing and manipulating textual data within applications.
What is a String?
A string in JavaScript is a series of characters, such as letters, numbers, and symbols, enclosed within single quotes (”) or double quotes (“”). For example, “Hello, World!” is a string containing the phrase “Hello, World!”
How Node.js Handles Strings
Node.js provides robust support for working with strings. Developers can perform various operations on strings, including concatenation, manipulation, and searching for specific words or characters within them.
The Magic of the includes() Method
One powerful method available for string manipulation in JavaScript is the includes() method. This method allows developers to check whether a string contains a specific word or character sequence.
Syntax and How To Use includes()
The syntax for the includes() method is straightforward:
const str = "Hello, World!";
const wordToFind = "World";
const containsWord = str.includes(wordToFind);
In this example, containsWord will be true since the string “Hello, World!” indeed contains the word “World”.
Case Sensitivity in String Search
It’s important to note that the includes() method performs a case-sensitive search by default. This means that it distinguishes between uppercase and lowercase letters when checking for the presence of a specific word or character sequence within a string.
The includes() function can also be used with strings to search for the presence of the specified string or character in the given string. It returns true if the specified string or character is found and false otherwise.
Practical Examples and Tips
Now that we understand how the includes() method works, let’s explore some practical examples and tips for effectively using it to check if a string contains a specific word in Node.js.
Real-World Use Case: Filtering User Input
When building applications that involve user-generated content, it’s crucial to implement robust input filtering mechanisms. One common scenario is checking for forbidden words within user input.
Checking for Forbidden Words
By utilizing the includes() method, developers can easily check whether user input contains any forbidden words. This ensures that the content meets specific guidelines and maintains a safe and respectful environment for all users.
Implementing a Simple Content Filter
Developers can implement a simple content filter by creating an array of forbidden words and then iterating through user input to check for their presence. If any forbidden words are found, appropriate actions can be taken to handle the situation gracefully.
Enhancing Your String Search
To further enhance your string search capabilities, consider combining the includes() method with other string manipulation methods available in JavaScript.
Combining includes() with Other String Methods
One powerful approach is combining includes() with methods like toLowerCase() or toUpperCase() to perform case-insensitive searches. This ensures that the search is not affected by variations in letter casing, providing more comprehensive results.
Another useful combination is using includes() alongside replace() to dynamically modify strings based on specific word occurrences. This allows for dynamic content manipulation based on the presence of particular words within a string.
Handling Edge Cases and Errors
It’s essential to consider edge cases and potential errors when using the includes() method. For instance, when searching for multi-word phrases, developers should account for scenarios where partial matches might occur. Additionally, error handling should be implemented to gracefully manage situations where unexpected data types are encountered during the search process.
By incorporating these tips and examples into your Node.js projects, you can effectively leverage the power of the includes() method to enhance string search functionality while ensuring robust input filtering mechanisms.
Wrapping Up
As we conclude our exploration of checking for specific words in Node.js strings, it’s essential to reflect on key takeaways and identify further learning resources to continue expanding your knowledge.
Key Takeaways
- The includes() method in JavaScript provides a powerful tool for efficiently checking if a string contains a specific word or character sequence. Its straightforward syntax and case-sensitive nature make it an invaluable asset for string manipulation tasks.
- When implementing input filtering mechanisms in Node.js applications, leveraging the includes() method can significantly enhance the security and integrity of user-generated content. By checking for forbidden words and implementing content filters, developers can maintain a safe and respectful environment within their applications.
- Combining the includes() method with other string manipulation methods, such as toLowerCase() or toUpperCase(), allows for more comprehensive and flexible string search capabilities. Additionally, handling edge cases and errors is crucial to ensure robust functionality when using the includes() method.
Further Learning Resources
Official Node.js Documentation
For in-depth learning and reference, the official Node.js documentation serves as a comprehensive resource covering core concepts, APIs, and best practices. It provides detailed insights into utilizing Node.js effectively for various development scenarios.
Online JavaScript Communities
Engaging with online JavaScript communities offers valuable opportunities to connect with fellow developers, seek advice, and stay updated on the latest trends and best practices in JavaScript and Node.js development. Platforms like Stack Overflow, GitHub discussions, and Reddit communities provide vibrant spaces for learning and sharing knowledge.
By continuing to explore these resources, you can further solidify your understanding of string manipulation in Node.js while staying connected with the broader developer community.